Build a Real-World Project Using JavaScript
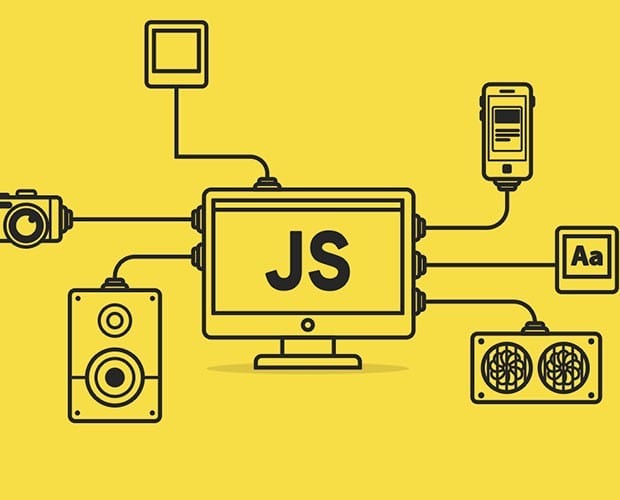
What’s included
$14.99 / $24.99
Get ready for your exam by enrolling in our comprehensive training course. This course includes a full set of instructional videos designed to equip you with in-depth knowledge essential for passing the certification exam with flying colors.
Pay once, own it forever
Video Courses
Course Introduction
Lectures | Duration |
---|---|
1. Setting up Our Tools: Brackets and Google Chrome | 3m 48s |
1. Setting up Our Tools: Brackets and Google Chrome
3m 48s
JavaScript Language Basics
Lectures | Duration |
---|---|
1. Section Intro | 49s |
2. Introduction to JavaScript | 4m 7s |
3. Getting Started with JavaScript | 4m 24s |
4. Variables and Data Types | 8m 43s |
5. Variable Mutation and Type Coercion | 15m 13s |
6. Operators | 13m 57s |
7. If / else Statements | 8m 56s |
8. Boolean Logic and Switch Statements | 15m 48s |
9. Coding Challenge 1 | 1m 39s |
10. Coding Challenge 1: Solution | 13m 51s |
11. Functions | 15m 31s |
12. Statements and Expressions | 2m 44s |
13. Arrays | 11m 10s |
14. Objects and Properties | 9m |
15. Objects and Methods | 14m 55s |
16. Loops and Iteration | 19m 6s |
17. Coding Challenge 2 | 2m 52s |
18. Coding Challenge 2: Solution | 13m 7s |
19. Important Note: ES5, ES6 / ES2015 and ES2016 | 4m 47s |
1. Section Intro
49s
2. Introduction to JavaScript
4m 7s
3. Getting Started with JavaScript
4m 24s
4. Variables and Data Types
8m 43s
5. Variable Mutation and Type Coercion
15m 13s
6. Operators
13m 57s
7. If / else Statements
8m 56s
8. Boolean Logic and Switch Statements
15m 48s
9. Coding Challenge 1
1m 39s
10. Coding Challenge 1: Solution
13m 51s
11. Functions
15m 31s
12. Statements and Expressions
2m 44s
13. Arrays
11m 10s
14. Objects and Properties
9m
15. Objects and Methods
14m 55s
16. Loops and Iteration
19m 6s
17. Coding Challenge 2
2m 52s
18. Coding Challenge 2: Solution
13m 7s
19. Important Note: ES5, ES6 / ES2015 and ES2016
4m 47s
How JavaScript Works Behind the Scenes
Lectures | Duration |
---|---|
1. Section Intro | 1m 7s |
2. How Our Code Is Executed: JavaScript Parsers and Engines | 2m 33s |
3. Execution Contexts and the Execution Stack | 5m 38s |
4. Execution Contexts in Detail: Creation and Execution Phases and Hoisting | 4m 5s |
5. Hoisting in Practice | 12m 6s |
6. Scoping and the Scope Chain | 12m 44s |
7. The 'this' Keyword | 1m 38s |
8. The 'this' Keyword in Practice | 11m 11s |
1. Section Intro
1m 7s
2. How Our Code Is Executed: JavaScript Parsers and Engines
2m 33s
3. Execution Contexts and the Execution Stack
5m 38s
4. Execution Contexts in Detail: Creation and Execution Phases and Hoisting
4m 5s
5. Hoisting in Practice
12m 6s
6. Scoping and the Scope Chain
12m 44s
7. The 'this' Keyword
1m 38s
8. The 'this' Keyword in Practice
11m 11s
JavaScript in the Browser: DOM Manipulation and Events
Lectures | Duration |
---|---|
1. Section Intro | 40s |
2. The DOM and DOM Manipulation | 1m 47s |
3. 5-Minute HTML and CSS Crash Course | 5m 6s |
4. Project Setup and Details | 4m 8s |
5. First DOM Access and Manipulation | 19m 33s |
6. Events and Event Handling: Rolling the Dice | 17m 17s |
7. Updating Scores and Changing the Active Player | 13m 54s |
8. Implementing Our 'Hold' Function and the DRY Principle | 17m 11s |
9. Creating a Game Initialization Function | 11m 10s |
10. Finishing Touches: State Variables | 7m 30s |
11. Coding Challenge 3 | 2m 12s |
12. Coding Challenge 3: Solution, Part 1 | 6m 3s |
13. Coding Challenge 3: Solution, Part 2 | 8m 51s |
14. Coding Challenge 3: Solution, Part 3 | 6m 56s |
1. Section Intro
40s
2. The DOM and DOM Manipulation
1m 47s
3. 5-Minute HTML and CSS Crash Course
5m 6s
4. Project Setup and Details
4m 8s
5. First DOM Access and Manipulation
19m 33s
6. Events and Event Handling: Rolling the Dice
17m 17s
7. Updating Scores and Changing the Active Player
13m 54s
8. Implementing Our 'Hold' Function and the DRY Principle
17m 11s
9. Creating a Game Initialization Function
11m 10s
10. Finishing Touches: State Variables
7m 30s
11. Coding Challenge 3
2m 12s
12. Coding Challenge 3: Solution, Part 1
6m 3s
13. Coding Challenge 3: Solution, Part 2
8m 51s
14. Coding Challenge 3: Solution, Part 3
6m 56s
Advanced JavaScript: Objects and Functions
Lectures | Duration |
---|---|
1. Section Intro | 1m 2s |
2. Everything Is an Object: Inheritance and the Prototype Chain | 9m 45s |
3. Creating Objects: Function Constructors | 14m 6s |
4. The Prototype Chain in the Console | 8m 19s |
5. Creating Objects: Object.create | 6m 26s |
6. Primitives vs | 8m 11s |
7. First Class Functions: Passing Functions as Arguments | 14m 44s |
8. First Class Functions: Functions Returning Functions | 8m 27s |
9. Immediately Invoked Function Expressions (IIFE) | 6m 19s |
10. Closures | 16m 24s |
11. Bind, Call and Apply | 16m 44s |
12. Coding Challenge 4 | 6m 49s |
13. Coding Challenge 4: Solution, Part 1 | 17m 47s |
14. Coding Challenge 4: Solution, Part 2 | 15m 46s |
1. Section Intro
1m 2s
2. Everything Is an Object: Inheritance and the Prototype Chain
9m 45s
3. Creating Objects: Function Constructors
14m 6s
4. The Prototype Chain in the Console
8m 19s
5. Creating Objects: Object.create
6m 26s
6. Primitives vs
8m 11s
7. First Class Functions: Passing Functions as Arguments
14m 44s
8. First Class Functions: Functions Returning Functions
8m 27s
9. Immediately Invoked Function Expressions (IIFE)
6m 19s
10. Closures
16m 24s
11. Bind, Call and Apply
16m 44s
12. Coding Challenge 4
6m 49s
13. Coding Challenge 4: Solution, Part 1
17m 47s
14. Coding Challenge 4: Solution, Part 2
15m 46s
Putting It All Together: The Budget App Project
Lectures | Duration |
---|---|
1. Section Intro | 1m 11s |
2. Project Setup and Details | 4m 26s |
3. Project Planning and Architecture: Step 1 | 5m 17s |
4. Implementing the Module Pattern | 17m 12s |
5. Setting up the First Event Listeners | 15m 43s |
6. Reading Input Data | 16m 10s |
7. Creating an Initialization Function | 5m 9s |
8. Creating Income and Expense Function Constructors | 9m 21s |
9. Adding a New Item to Our Budget Controller | 18m 2s |
10. Adding a New Item to the UI | 19m 28s |
11. Clearing Our Input Fields | 11m 23s |
12. Updating the Budget: Controller | 10m 54s |
13. Updating the Budget: Budget Controller | 20m 38s |
14. Updating the Budget: UI Controller | 11m 41s |
15. Project Planning and Architecture: Step 2 | 2m 16s |
16. Event Delegation | 3m 36s |
17. Setting up the Delete Event Listener Using Event Delegation | 19m 13s |
18. Deleting an Item from Our Budget Controller | 17m 9s |
19. Deleting an Item from the UI | 7m 51s |
20. Project Planning and Architecture: Step 3 | 1m 46s |
21. Updating the Percentages: Controller | 3m 37s |
22. Updating the Percentages: Budget Controller | 14m 21s |
23. Updating the Percentages: UI Controller | 11m 49s |
24. Formatting Our Budget Numbers: String Manipulation | 19m |
25. Displaying the Current Month and Year | 6m 48s |
26. Finishing Touches: Improving the UX | 10m 17s |
27. We’ve Made It! Final Considerations | 1m 10s |
1. Section Intro
1m 11s
2. Project Setup and Details
4m 26s
3. Project Planning and Architecture: Step 1
5m 17s
4. Implementing the Module Pattern
17m 12s
5. Setting up the First Event Listeners
15m 43s
6. Reading Input Data
16m 10s
7. Creating an Initialization Function
5m 9s
8. Creating Income and Expense Function Constructors
9m 21s
9. Adding a New Item to Our Budget Controller
18m 2s
10. Adding a New Item to the UI
19m 28s
11. Clearing Our Input Fields
11m 23s
12. Updating the Budget: Controller
10m 54s
13. Updating the Budget: Budget Controller
20m 38s
14. Updating the Budget: UI Controller
11m 41s
15. Project Planning and Architecture: Step 2
2m 16s
16. Event Delegation
3m 36s
17. Setting up the Delete Event Listener Using Event Delegation
19m 13s
18. Deleting an Item from Our Budget Controller
17m 9s
19. Deleting an Item from the UI
7m 51s
20. Project Planning and Architecture: Step 3
1m 46s
21. Updating the Percentages: Controller
3m 37s
22. Updating the Percentages: Budget Controller
14m 21s
23. Updating the Percentages: UI Controller
11m 49s
24. Formatting Our Budget Numbers: String Manipulation
19m
25. Displaying the Current Month and Year
6m 48s
26. Finishing Touches: Improving the UX
10m 17s
27. We’ve Made It! Final Considerations
1m 10s
Get Ready for the Future: ES6 / ES2015
Lectures | Duration |
---|---|
1. What's new in ES6 / ES2015 | 4m 43s |
2. Variable Declarations with let and const | 16m 17s |
3. Blocks and IIFEs | 3m 38s |
4. Strings in ES6 / ES2015 | 10m 2s |
5. Arrow Functions: Basics | 8m 4s |
6. Arrow Functions: Lexical 'this' Keyword | 19m 11s |
7. Destructuring | 7m 51s |
8. Arrays in ES6 / ES2015 | 17m 19s |
9. The Spread Operator | 9m 56s |
10. Rest Parameters | 13m 23s |
11. Default Parameters | 7m 48s |
12. Maps | 19m 53s |
13. Classes | 9m 47s |
14. Classes with Subclasses | 15m 47s |
15. Coding Challenge 5 | 2m 46s |
16. Coding Challenge 5: Solution | 30m 58s |
17. How to use ES2015 / ES6 Today! | 14m 9s |
1. What's new in ES6 / ES2015
4m 43s
2. Variable Declarations with let and const
16m 17s
3. Blocks and IIFEs
3m 38s
4. Strings in ES6 / ES2015
10m 2s
5. Arrow Functions: Basics
8m 4s
6. Arrow Functions: Lexical 'this' Keyword
19m 11s
7. Destructuring
7m 51s
8. Arrays in ES6 / ES2015
17m 19s
9. The Spread Operator
9m 56s
10. Rest Parameters
13m 23s
11. Default Parameters
7m 48s
12. Maps
19m 53s
13. Classes
9m 47s
14. Classes with Subclasses
15m 47s
15. Coding Challenge 5
2m 46s
16. Coding Challenge 5: Solution
30m 58s
17. How to use ES2015 / ES6 Today!
14m 9s
Conclusion
Lectures | Duration |
---|---|
1. Where to Go from Here | 1m 45s |
1. Where to Go from Here
1m 45s