1z0-809: Java SE 8 Programmer II
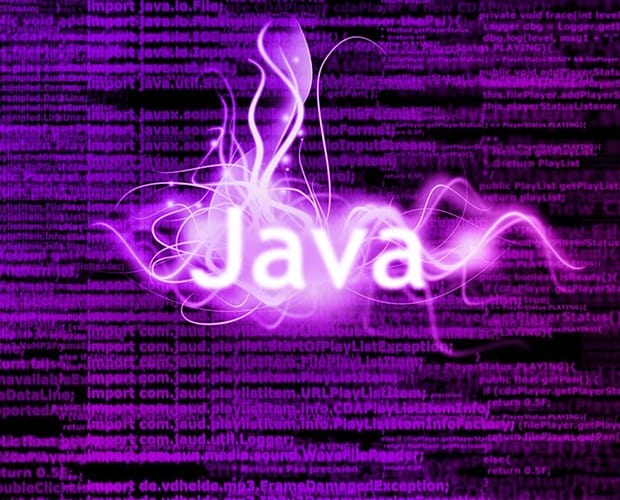
What’s included
$14.99 / $24.99
Get ready for your exam by enrolling in our comprehensive training course. This course includes a full set of instructional videos designed to equip you with in-depth knowledge essential for passing the certification exam with flying colors.
Pay once, own it forever
Video Courses
Java Class Design
Lectures | Duration |
---|---|
1. Introduction | 1m 34s |
2. 1.1 Implement Encapsulation | 6m 32s |
3. 1.2 Implement inheritance including visibility modifiers and composition | 5m 55s |
4. 1.3 Implement Polymorphism | 5m 15s |
5. 1.4 Override hashCode, equals, and toString methods from Object class | 10m 21s |
6. 1.5 Create and use singleton classes and immutable classes | 12m 56s |
7. 1.6 Develop code that uses static keyword on initialize blocks, variables, metho | 10m 18s |
1. Introduction
1m 34s
2. 1.1 Implement Encapsulation
6m 32s
3. 1.2 Implement inheritance including visibility modifiers and composition
5m 55s
4. 1.3 Implement Polymorphism
5m 15s
5. 1.4 Override hashCode, equals, and toString methods from Object class
10m 21s
6. 1.5 Create and use singleton classes and immutable classes
12m 56s
7. 1.6 Develop code that uses static keyword on initialize blocks, variables, metho
10m 18s
Advanced Java Class Design
Lectures | Duration |
---|---|
1. 2.1 Develop code that uses abstract classes and methods | 7m 7s |
2. 2.2 Develop code that uses the final keyword | 9m |
3. 2.3 Create inner classes including static inner class, local class, nested class | 14m 41s |
4. 2.4 Use enumerated types including methods, and constructors in an enum type | 13m 56s |
5. 2.5 Develop code that declares, implements and/or extends interfaces and use the | 16m 41s |
6. 2.6 Create and use Lambda expressions | 14m 1s |
1. 2.1 Develop code that uses abstract classes and methods
7m 7s
2. 2.2 Develop code that uses the final keyword
9m
3. 2.3 Create inner classes including static inner class, local class, nested class
14m 41s
4. 2.4 Use enumerated types including methods, and constructors in an enum type
13m 56s
5. 2.5 Develop code that declares, implements and/or extends interfaces and use the
16m 41s
6. 2.6 Create and use Lambda expressions
14m 1s
Generics and Collections
Lectures | Duration |
---|---|
1. 3.1 Create and use a generic class | 14m 50s |
2. 3.2 Create and use ArrayList, TreeSet, TreeMap, and ArrayDeque objects | 31m 27s |
3. 3.3 Use java.util.Comparator and java.lang.Comparable interfaces | 17m 12s |
4. 3.4 Collections Streams and Filters | 8m 56s |
5. 3.5 Iterate using forEach methods of Streams and List | 7m 59s |
6. 3.6 Describe Stream interface and Stream pipeline | 10m 21s |
7. 3.7 Filter a collection by using lambda expressions | 5m 37s |
8. 3.8 Use method references with Streams | 14m 1s |
1. 3.1 Create and use a generic class
14m 50s
2. 3.2 Create and use ArrayList, TreeSet, TreeMap, and ArrayDeque objects
31m 27s
3. 3.3 Use java.util.Comparator and java.lang.Comparable interfaces
17m 12s
4. 3.4 Collections Streams and Filters
8m 56s
5. 3.5 Iterate using forEach methods of Streams and List
7m 59s
6. 3.6 Describe Stream interface and Stream pipeline
10m 21s
7. 3.7 Filter a collection by using lambda expressions
5m 37s
8. 3.8 Use method references with Streams
14m 1s
Lambda Built-in Functional Interfaces
Lectures | Duration |
---|---|
1. 4.1 Use the built-in interfaces included in the java.util.function package such | 16m 49s |
2. 4.2 Develop code that uses primitive versions of functional interfaces | 16m 2s |
3. 4.3 Develop code that uses binary versions of functional interfaces | 13m 59s |
4. 4.4 Develop code that uses the UnaryOperator interface | 4m 55s |
1. 4.1 Use the built-in interfaces included in the java.util.function package such
16m 49s
2. 4.2 Develop code that uses primitive versions of functional interfaces
16m 2s
3. 4.3 Develop code that uses binary versions of functional interfaces
13m 59s
4. 4.4 Develop code that uses the UnaryOperator interface
4m 55s
Java Stream API
Lectures | Duration |
---|---|
1. 5.1 Develop code to extract data from an object using peek() and map() methods i | 8m 57s |
2. 5.2 Search for data by using search methods of the Stream classes including find | 11m 55s |
3. 5.3 Develop code that uses the Optional class | 20m 51s |
4. 5.4 Develop code that uses Stream data methods and calculation methods | 14m 23s |
5. 5.5 Sort a collection using Stream API | 7m 12s |
6. 5.6 Save results to a collection using the collect method and group/partition da | 20m 50s |
7. 5.7 Use flatMap() methods in the Stream API | 7m 59s |
1. 5.1 Develop code to extract data from an object using peek() and map() methods i
8m 57s
2. 5.2 Search for data by using search methods of the Stream classes including find
11m 55s
3. 5.3 Develop code that uses the Optional class
20m 51s
4. 5.4 Develop code that uses Stream data methods and calculation methods
14m 23s
5. 5.5 Sort a collection using Stream API
7m 12s
6. 5.6 Save results to a collection using the collect method and group/partition da
20m 50s
7. 5.7 Use flatMap() methods in the Stream API
7m 59s
Exceptions and Assertions
Lectures | Duration |
---|---|
1. 6.1 Use try-catch and throw statements | 7m 43s |
2. 6.2 Use catch, multi-catch, and finally clauses | 7m 32s |
3. 6.3 Use Autoclose resources with a try-with-resources statement | 10m 11s |
4. 6.4 Create custom exceptions and Auto-closeable resources | 11m 33s |
5. 6.5 Test invariants by using assertions | 12m 57s |
1. 6.1 Use try-catch and throw statements
7m 43s
2. 6.2 Use catch, multi-catch, and finally clauses
7m 32s
3. 6.3 Use Autoclose resources with a try-with-resources statement
10m 11s
4. 6.4 Create custom exceptions and Auto-closeable resources
11m 33s
5. 6.5 Test invariants by using assertions
12m 57s
Use Java SE 8 Date/Time API
Lectures | Duration |
---|---|
1. 7.1 Create and manage date-based and time-based events | 17m 18s |
2. 7.2 Work with dates and times across timezones and manage changes resulting from | 22m 12s |
3. 7.3 Define and create and manage date-based and time-based events using Instant, | 8m 37s |
1. 7.1 Create and manage date-based and time-based events
17m 18s
2. 7.2 Work with dates and times across timezones and manage changes resulting from
22m 12s
3. 7.3 Define and create and manage date-based and time-based events using Instant,
8m 37s
Java I/O Fundamentals
Lectures | Duration |
---|---|
1. 8.1 Read and write data from the console | 11m 41s |
2. 8.2 Use BufferedReader, BufferedWriter, File, FileReader and others in the java. | 21m 19s |
1. 8.1 Read and write data from the console
11m 41s
2. 8.2 Use BufferedReader, BufferedWriter, File, FileReader and others in the java.
21m 19s
Java File I/O (NIO.2)
Lectures | Duration |
---|---|
1. 9.1 Use Path interface to operate on file and directory paths | 16m 55s |
2. 9.2 Use Files class to check, read, delete, copy, move, manage metadata of a fil | 22m 5s |
3. 9.3 Use Stream API with NIO.2 | 11m 27s |
1. 9.1 Use Path interface to operate on file and directory paths
16m 55s
2. 9.2 Use Files class to check, read, delete, copy, move, manage metadata of a fil
22m 5s
3. 9.3 Use Stream API with NIO.2
11m 27s
Java Concurrency
Lectures | Duration |
---|---|
1. 10.1 Create worker threads using Runnable, Callable and use an ExecutorService t | 13m 36s |
2. 10.2 Identify potential threading problems among deadlock, starvation, livelock, | 13m 58s |
3. 10.3 Use synchronized keyword and java.util.concurrent.atomic package to control | 20m 57s |
4. 10.4 Use java.util.concurrent collections and classes including CyclicBarrier an | 12m 44s |
5. 10.5 Use parallel Fork/Join Framework | 12m 40s |
6. 10.6 Use parallel Streams including reduction, decomposition, merging processes | 11m 18s |
1. 10.1 Create worker threads using Runnable, Callable and use an ExecutorService t
13m 36s
2. 10.2 Identify potential threading problems among deadlock, starvation, livelock,
13m 58s
3. 10.3 Use synchronized keyword and java.util.concurrent.atomic package to control
20m 57s
4. 10.4 Use java.util.concurrent collections and classes including CyclicBarrier an
12m 44s
5. 10.5 Use parallel Fork/Join Framework
12m 40s
6. 10.6 Use parallel Streams including reduction, decomposition, merging processes
11m 18s
Building Database Applications with JDBC
Lectures | Duration |
---|---|
1. 11.2 Identify the components required to connect to a database using the DriverM | 9m 47s |
2. 11.1 Describe the interfaces that make up the core of the JDBC API including the | 6m 33s |
3. 11.3 Submit queries and read results from the database | 10m 46s |
1. 11.2 Identify the components required to connect to a database using the DriverM
9m 47s
2. 11.1 Describe the interfaces that make up the core of the JDBC API including the
6m 33s
3. 11.3 Submit queries and read results from the database
10m 46s
Localization
Lectures | Duration |
---|---|
1. 12.1 Read and set the locale by using the Locale object | 10m 27s |
2. 12.2 Create and read a Properties file | 7m 26s |
3. 12.3 Build a resource bundle for each locale and load a resource bundle in an ap | 10m 49s |
1. 12.1 Read and set the locale by using the Locale object
10m 27s
2. 12.2 Create and read a Properties file
7m 26s
3. 12.3 Build a resource bundle for each locale and load a resource bundle in an ap
10m 49s