Golang Comprehensive Course
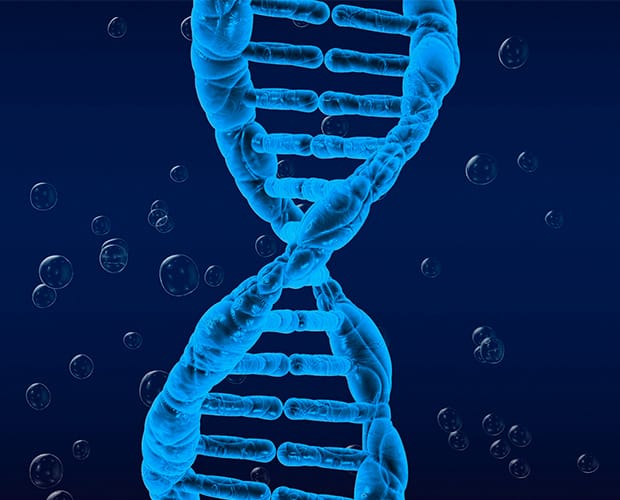
What’s included
$14.99 / $24.99
Get ready for your exam by enrolling in our comprehensive training course. This course includes a full set of instructional videos designed to equip you with in-depth knowledge essential for passing the certification exam with flying colors.
Pay once, own it forever
Video Courses
Getting Started
Lectures | Duration |
---|---|
1. Environment Setup | 3m |
2. VSCode Installation | 3m |
3. Go Support in VSCode | 3m |
1. Environment Setup
3m
2. VSCode Installation
3m
3. Go Support in VSCode
3m
A Simple Start
Lectures | Duration |
---|---|
1. Boring Ol' Hello World | 3m |
2. Five Important Questions | 6m |
3. Go Packages | 6m |
4. Import Statements | 4m |
5. File Organization | 4m |
1. Boring Ol' Hello World
3m
2. Five Important Questions
6m
3. Go Packages
6m
4. Import Statements
4m
5. File Organization
4m
Deeper Into Go
Lectures | Duration |
---|---|
1. Project Overview | 3m |
2. New Project Folder | 2m |
3. Variable Declarations | 11m |
4. Functions and Return Types | 8m |
5. Slices and For Loops | 11m |
6. OO Approach vs Go Approach | 5m |
7. Custom Type Declarations | 7m |
8. Receiver Functions | 6m |
9. Creating a New Deck | 11m |
10. Slice Range Syntax | 6m |
11. Multiple Return Values | 8m |
12. Byte Slices | 7m |
13. Deck to String | 7m |
14. Joining a Slice of Strings | 7m |
15. Saving Data to the Hard Drive | 7m |
16. Reading From the Hard Drive | 11m |
17. Error Handling | 8m |
18. Shuffling a Deck | 10m |
19. Random Number Generation | 11m |
20. Testing With Go | 4m |
21. Writing Useful Tests | 12m |
22. Asserting Elements in a Slice | 4m |
23. Testing File IO | 10m |
24. Project Review | 6m |
1. Project Overview
3m
2. New Project Folder
2m
3. Variable Declarations
11m
4. Functions and Return Types
8m
5. Slices and For Loops
11m
6. OO Approach vs Go Approach
5m
7. Custom Type Declarations
7m
8. Receiver Functions
6m
9. Creating a New Deck
11m
10. Slice Range Syntax
6m
11. Multiple Return Values
8m
12. Byte Slices
7m
13. Deck to String
7m
14. Joining a Slice of Strings
7m
15. Saving Data to the Hard Drive
7m
16. Reading From the Hard Drive
11m
17. Error Handling
8m
18. Shuffling a Deck
10m
19. Random Number Generation
11m
20. Testing With Go
4m
21. Writing Useful Tests
12m
22. Asserting Elements in a Slice
4m
23. Testing File IO
10m
24. Project Review
6m
Organizing Data With Structs
Lectures | Duration |
---|---|
1. Structs in Go | 5m |
2. Defining Structs | 4m |
3. Declaring Structs | 5m |
4. Updating Struct Values | 6m |
5. Embedding Structs | 7m |
6. Structs with Receiver Functions | 7m |
7. Pass By Value | 6m |
8. Structs with Pointers | 3m |
9. Pointer Operations | 10m |
10. Pointer Shortcut | 6m |
11. Gotchas With Pointers | 4m |
12. Reference vs Value Types | 8m |
1. Structs in Go
5m
2. Defining Structs
4m
3. Declaring Structs
5m
4. Updating Struct Values
6m
5. Embedding Structs
7m
6. Structs with Receiver Functions
7m
7. Pass By Value
6m
8. Structs with Pointers
3m
9. Pointer Operations
10m
10. Pointer Shortcut
6m
11. Gotchas With Pointers
4m
12. Reference vs Value Types
8m
Maps
Lectures | Duration |
---|---|
1. What's a Map? | 6m |
2. Manipulating Maps | 5m |
3. Iterating Over Maps | 5m |
4. Differences Between Maps and Structs | 6m |
1. What's a Map?
6m
2. Manipulating Maps
5m
3. Iterating Over Maps
5m
4. Differences Between Maps and Structs
6m
Interfaces
Lectures | Duration |
---|---|
1. Purpose of Interfaces | 9m |
2. Problems Without Interfaces | 10m |
3. Interfaces in Practice | 9m |
4. Rules of Interfaces | 8m |
5. Extra Interface Notes | 7m |
6. The HTTP Package | 8m |
7. Reading the Docs | 6m |
8. More Interface Syntax | 3m |
9. Interface Review | 2m |
10. The Reader Interface | 8m |
11. More on the Reader Interface | 7m |
12. Working with the Read Function | 6m |
13. The Writer Interface | 4m |
14. The io.Copy Function | 5m |
15. The Implementation of io.Copy | 5m |
16. A Custom Writer | 8m |
1. Purpose of Interfaces
9m
2. Problems Without Interfaces
10m
3. Interfaces in Practice
9m
4. Rules of Interfaces
8m
5. Extra Interface Notes
7m
6. The HTTP Package
8m
7. Reading the Docs
6m
8. More Interface Syntax
3m
9. Interface Review
2m
10. The Reader Interface
8m
11. More on the Reader Interface
7m
12. Working with the Read Function
6m
13. The Writer Interface
4m
14. The io.Copy Function
5m
15. The Implementation of io.Copy
5m
16. A Custom Writer
8m
Channels and Go Routines
Lectures | Duration |
---|---|
1. Website Status Checker | 5m |
2. Printing Site Status | 5m |
3. Serial Link Checking | 3m |
4. Go Routines | 7m |
5. Theory of Go Routines | 9m |
6. Channels | 6m |
7. Channel Implementation | 9m |
8. Blocking Channels | 10m |
9. Receiving Messages | 4m |
10. Repeating Routines | 7m |
11. Alternative Loop Syntax | 4m |
12. Sleeping a Routine | 6m |
13. Function Literals | 5m |
14. Channels Gotcha! | 11m |
1. Website Status Checker
5m
2. Printing Site Status
5m
3. Serial Link Checking
3m
4. Go Routines
7m
5. Theory of Go Routines
9m
6. Channels
6m
7. Channel Implementation
9m
8. Blocking Channels
10m
9. Receiving Messages
4m
10. Repeating Routines
7m
11. Alternative Loop Syntax
4m
12. Sleeping a Routine
6m
13. Function Literals
5m
14. Channels Gotcha!
11m